Introduction
Ajax Helpers make simple to create AJAX enabled elements like as Ajax enabled forms and links. Ajax Helpers are extension methods of AjaxHelper class. In this article we will see how these ajax helper works spacially following
- Ajax.BeginForm()
- Ajax.ActionLink()
DownLoad source code
You can downlad source code click here. You can run by providing Data Source in web.config file as
<connectionStrings>
<add name="AjaxHelperInMVCContext" connectionString="Data Source=SQLServerName; Initial Catalog=AjaxHelperInMVCContext-20160613184906; Integrated Security=True; MultipleActiveResultSets=True; AttachDbFilename=|DataDirectory|AjaxHelperInMVCContext-20160613184906.mdf" providerName="System.Data.SqlClient" />
</connectionStrings>
Ensure following before using Ajax Helpers
In web.config keys 'ClientValidationEnabled' and 'UnobtrusiveJavaScriptEnabled' should be true as
<appSettings>
<add key="webpages:Version" value="3.0.0.0" />
<add key="webpages:Enabled" value="false" />
<add key="ClientValidationEnabled" value="true" />
<add key="UnobtrusiveJavaScriptEnabled" value="true" />
</appSettings>
Following Js files
- jquery-{versions}.js
- jquery.unobtrusive-ajax.js
- jquery.validate.unobtrusive.js
should be included in the view. If not, install from nuget package manager.
Prerequisites
- Visual Studio 2015
- Code First Approach with Entity Framework 6
- Basic knowledge of MVC
Example
In this example I am try to create CRUD operation by using Ajax Helpers i.e. asynchronous functionality. First we create CRUD functionality by using scaffolding feature of ASP.NET and then modify it with appropriate Ajax Helpers. Follow the steps:
I) Create new web project with MVC template named 'AjaxHelperInMVC'
II) Create a Model class of Player as
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.ComponentModel.DataAnnotations;
namespace AjaxHelperInMVC.Models {
public class Player {
public int PlayerID { get; set; }
[Required]
public string PlayerName { get; set; }
public string Gender { get; set; }
}
}
III) Now create a controller class 'PlayersAjax' by using scaffolding as
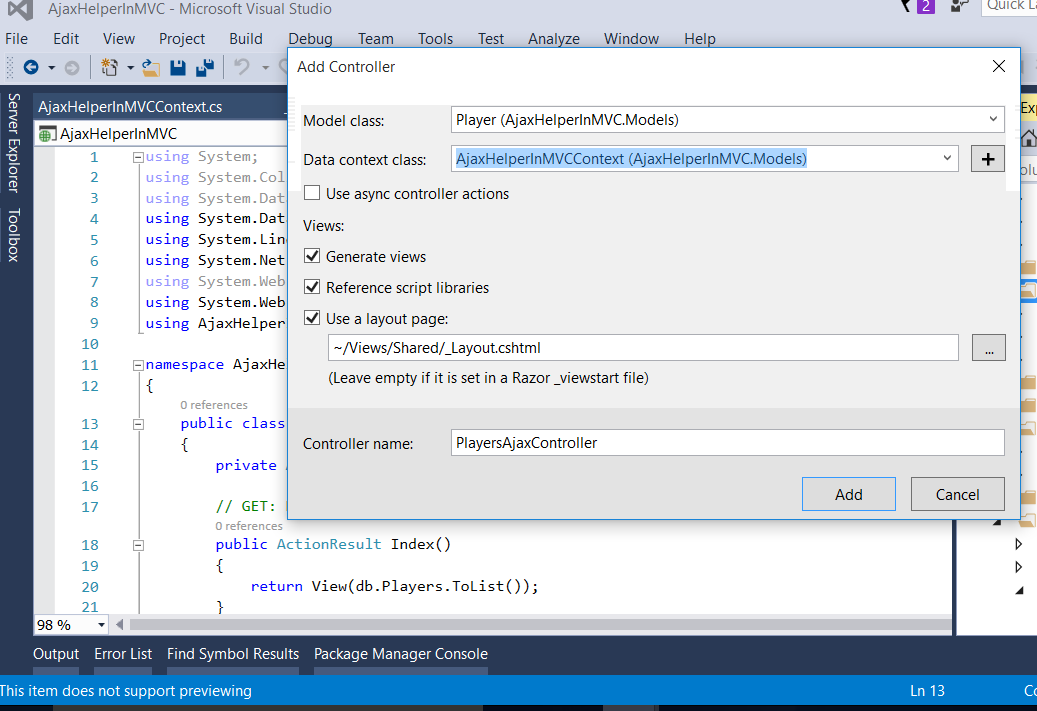
It will create list, add, edit and delete with normal HtmlHelper. You can see in Controller and views
IV) Now open view 'Index.cshtml' and modify Html.ActionLink to Ajax.ActionLink and for deletion use Ajax.BeginForm as
@model IEnumerable<AjaxHelperInMVC.Models.Player>
@{
ViewBag.Title = "Player";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Player</h2>
<p>
@Ajax.ActionLink("Create New", "Create",new AjaxOptions {UpdateTargetId= "dvCreateAjaxForm", HttpMethod="get" })
</p>
<div id="dvCreateAjaxForm">
</div>
<div id="dvPlayerList">
<table class="table">
<tr>
<th>
@Html.DisplayNameFor(model => model.PlayerName)
</th>
<th>
@Html.DisplayNameFor(model => model.Gender)
</th>
<th></th>
</tr>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.PlayerName)
</td>
<td>
@Html.DisplayFor(modelItem => item.Gender)
</td>
<td>
<table>
<tr>
<td>
@Ajax.ActionLink("Edit", "Edit", "PlayersAjax", new { id = item.PlayerID },new AjaxOptions { UpdateTargetId = "dvCreateAjaxForm", HttpMethod = "get" }, new { @class = "btn btn-default" })
</td>
<td>
@Ajax.ActionLink("Details", "Details", "PlayersAjax", new { id = item.PlayerID }, new AjaxOptions { UpdateTargetId = "dvCreateAjaxForm", HttpMethod = "get" }, new { @class = "btn btn-default" })
</td>
<td>
@using (Ajax.BeginForm("Delete", "PlayersAjax", new { id = item.PlayerID }, new AjaxOptions { OnBegin="ValidateDelete", UpdateTargetId = "dvPlayerList", HttpMethod = "post" })) {
@Html.AntiForgeryToken()
<input type="submit" value="Delete" class="btn btn-danger" />
}
</td>
</tr>
</table>
</td>
</tr>
}
</table>
</div>
<script>
function onBegin_Ajax(data) {
//code for Client validation
//progress bar code
$("#dvProgressbar").css("display", "");
}
function onComplete_Ajax(data) {
//code for close progress
}
function onSuccess_Ajax(data) {
//code for successfull ajax post
$("#dvCreateAjaxForm").html("");
$("#dvProgressbar").css("display", "none");
}
function onFailure_Ajax(data) {
//on failure show message
}
function ValidateDelete() {
return confirm("Are you sure?");
}
</script>
V) For create functionality open and modify 'Create.cshtml' as
@model AjaxHelperInMVC.Models.Player
@{
Layout = null;
}
<h2>Create</h2>
@*@using (Ajax.BeginForm("Create", new AjaxOptions { UpdateTargetId = "", HttpMethod = "post" }))*@
@using (Ajax.BeginForm("Create", "PlayersAjax", null, new AjaxOptions {
UpdateTargetId = "dvPlayerList",
OnBegin = "onBegin_Ajax",
OnComplete = "onComplete_Ajax",
OnSuccess = "onSuccess_Ajax",
OnFailure = "onFailure_Ajax",
HttpMethod = "post"
})) {
@Html.AntiForgeryToken()
<div class="form-horizontal">
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
<div class="form-group">
@Html.LabelFor(model => model.PlayerName, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.PlayerName, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.PlayerName, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Gender, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Gender, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Gender, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Create" class="btn btn-default" />
</div>
</div>
</div>
}
<div>
</div>
VI) Create a partial view to load list 'PlayerListPartialView.cshtml' as
@model IEnumerable<AjaxHelperInMVC.Models.Player>
<table class="table">
<tr>
<th>
@Html.DisplayNameFor(model => model.PlayerName)
</th>
<th>
@Html.DisplayNameFor(model => model.Gender)
</th>
<th></th>
</tr>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.PlayerName)
</td>
<td>
@Html.DisplayFor(modelItem => item.Gender)
</td>
<td>
<table>
<tr>
<td>
@Ajax.ActionLink("Edit", "Edit", "PlayersAjax", new { id = item.PlayerID }, new AjaxOptions { UpdateTargetId = "dvCreateAjaxForm", HttpMethod = "get" }, new { @class = "btn btn-default" })
</td>
<td>
@Ajax.ActionLink("Details", "Details", "PlayersAjax", new { id = item.PlayerID }, new AjaxOptions { UpdateTargetId = "dvCreateAjaxForm", HttpMethod = "get" }, new { @class = "btn btn-default" })
</td>
<td>
@using (Ajax.BeginForm("Delete", "PlayersAjax", new { id = item.PlayerID }, new AjaxOptions { OnBegin = "ValidateDelete", UpdateTargetId = "dvPlayerList", HttpMethod = "post" })) {
@Html.AntiForgeryToken()
<input type="submit" value="Delete" class="btn btn-danger" />
}
</td>
</tr>
</table>
</td>
</tr>
}
</table>
VII) Modify edit view 'Edit.cshtml' as
@model AjaxHelperInMVC.Models.Player
@{
Layout = null;
}
<h2>Edit</h2>
@*@using (Ajax.BeginForm("Create", new AjaxOptions { UpdateTargetId = "", HttpMethod = "post" }))*@
@using (Ajax.BeginForm("Edit", "PlayersAjax", null, new AjaxOptions {
UpdateTargetId = "dvPlayerList",
OnBegin = "onBegin_Ajax",
OnComplete = "onComplete_Ajax",
OnSuccess = "onSuccess_Ajax",
OnFailure = "onFailure_Ajax",
HttpMethod = "post"
})) {
@Html.AntiForgeryToken()
<div class="form-horizontal">
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
@Html.HiddenFor(model => model.PlayerID)
<div class="form-group">
@Html.LabelFor(model => model.PlayerName, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.PlayerName, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.PlayerName, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Gender, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Gender, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Gender, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Update" class="btn btn-default" />
</div>
</div>
</div>
}
VIII) Now some modification in controller 'PlayersAjax' as
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.Entity;
using System.Linq;
using System.Net;
using System.Web;
using System.Web.Mvc;
using AjaxHelperInMVC.Models;
namespace AjaxHelperInMVC.Controllers
{
public class PlayersAjaxController : Controller
{
private AjaxHelperInMVCContext db = new AjaxHelperInMVCContext();
// GET: PlayersAjax
public ActionResult Index()
{
return View(db.Players.ToList());
}
// GET: PlayersAjax/Details/5
public ActionResult Details(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Player player = db.Players.Find(id);
if (player == null)
{
return HttpNotFound();
}
return View(player);
}
// GET: PlayersAjax/Create
public ActionResult Create()
{
return PartialView();
}
// POST: PlayersAjax/Create
// To protect from overposting attacks, please enable the specific properties you want to bind to, for
// more details see https://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create([Bind(Include = "PlayerID,PlayerName,Gender")] Player player)
{
if (ModelState.IsValid)
{
System.Threading.Thread.Sleep(1000);
db.Players.Add(player);
db.SaveChanges();
}
return PartialView("PlayerListPartialView", db.Players.ToList());
}
// GET: PlayersAjax/Edit/5
public ActionResult Edit(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Player player = db.Players.Find(id);
if (player == null)
{
return HttpNotFound();
}
return PartialView(player);
}
// POST: PlayersAjax/Edit/5
// To protect from overposting attacks, please enable the specific properties you want to bind to, for
// more details see https://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Edit([Bind(Include = "PlayerID,PlayerName,Gender")] Player player)
{
if (ModelState.IsValid)
{
db.Entry(player).State = EntityState.Modified;
db.SaveChanges();
}
return PartialView("PlayerListPartialView", db.Players.ToList());
}
//// GET: PlayersAjax/Delete/5
//public ActionResult Delete(int? id)
//{
// if (id == null)
// {
// return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
// }
// Player player = db.Players.Find(id);
// if (player == null)
// {
// return HttpNotFound();
// }
// return View(player);
//}
// POST: PlayersAjax/Delete/5
[HttpPost,ActionName("Delete")]
[ValidateAntiForgeryToken]
public ActionResult DeleteConfirmed(int id)
{
Player player = db.Players.Find(id);
db.Players.Remove(player);
db.SaveChanges();
return PartialView("PlayerListPartialView", db.Players.ToList());
}
protected override void Dispose(bool disposing)
{
if (disposing)
{
db.Dispose();
}
base.Dispose(disposing);
}
}
}
IX) Add unobtrusive file in layout view as
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>@ViewBag.Title - My ASP.NET Application</title>
@Styles.Render("~/Content/css")
@Scripts.Render("~/bundles/modernizr")
</head>
<body>
<div class="navbar navbar-inverse navbar-fixed-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle" data-toggle="collapse" data-target=".navbar-collapse">
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
@Html.ActionLink("Application name", "Index", "Home", new { area = "" }, new { @class = "navbar-brand" })
</div>
<div class="navbar-collapse collapse">
<ul class="nav navbar-nav">
<li>@Html.ActionLink("Home", "Index", "Home")</li>
<li>@Html.ActionLink("About", "About", "Home")</li>
<li>@Html.ActionLink("Contact", "Contact", "Home")</li>
<li>@Html.ActionLink("Player", "Index", "Players")</li>
<li>@Html.ActionLink("Player Ajax", "Index", "PlayersAjax")</li>
</ul>
</div>
</div>
</div>
<div id="dvProgressbar" style="display:none;">Loading...</div>
<div class="container body-content">
@RenderBody()
<hr />
<footer>
<p>© @DateTime.Now.Year - My ASP.NET Application</p>
</footer>
</div>
@Scripts.Render("~/bundles/jquery")
@Scripts.Render("~/bundles/bootstrap")
@Scripts.Render("~/bundles/unobtrusive_ajax")
@RenderSection("scripts", required: false)
</body>
</html>
X) Now Build and run application in debug mode out put will be as
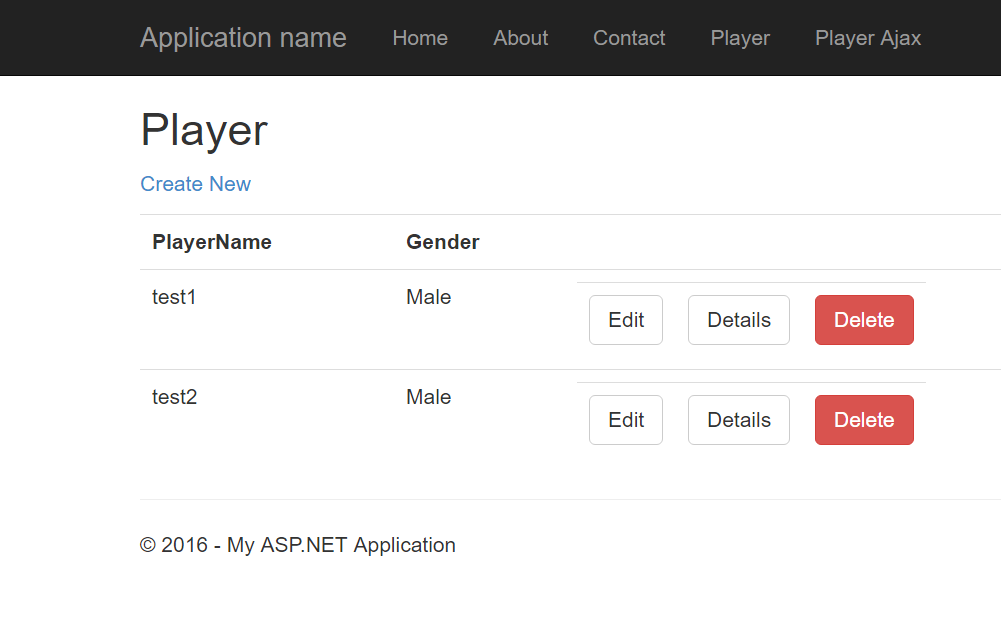
You can download this example click here and you can run by providing Data Source as
<connectionStrings>
<add name="AjaxHelperInMVCContext" connectionString="Data Source=SQLServerName; Initial Catalog=AjaxHelperInMVCContext-20160613184906; Integrated Security=True; MultipleActiveResultSets=True; AttachDbFilename=|DataDirectory|AjaxHelperInMVCContext-20160613184906.mdf" providerName="System.Data.SqlClient" />
</connectionStrings>
Conclusion
In above example we learn how to use AjaxHelper. I hope it will help you for using these helpers.
Rahul Kiwitech
28-Jul-2016 at 05:25